When it comes to developing complex enterprise applications, you want a platform that’s reliable, efficient, and cost-effective. Look no further than the Laravel framework, which offers the simplest and most preferred way to create web applications. There are numerous reasons why Laravel stands out as a top choice in web app development. In this article, we are going to discuss the top 10 Laravel best practices for creating quality SaaS applications.
Firstly, it’s free to use since it’s an open-source PHP framework. This means you can save on licensing costs and invest more in enhancing your application. Secondly, Laravel comes with a wide range of easily accessible and cutting-edge features. These include simpler verification processes, secure routing options, and the ability to conduct unit tests seamlessly.
All these advantages make Laravel highly desirable and achievable for developers looking to create powerful web applications without unnecessary complexities. Embrace Laravel and unlock its full potential for your web development projects.
Keep Laravel up to date
Staying current with Laravel offers a range of advantages that can greatly enhance your web development experience:
- Enhanced Security: Regular updates from Laravel ensure that security fixes are promptly implemented, safeguarding your application from potential vulnerabilities.
- Improved Performance: Laravel updates often come with performance enhancements, resulting in faster load times and more efficient code execution, ultimately improving the overall user experience.
- New Features and Functionality: Embracing the updates allows you to access and enjoy the latest features and functionalities introduced by Laravel, empowering you to create more powerful and feature-rich applications.
- Compatibility with Official and Community Packages: By keeping up with the updates, you ensure that your Laravel application remains compatible with the latest official and community packages, facilitating seamless integration of external tools and extensions.
If the idea of Laravel updates makes you apprehensive, it might be a sign that your codebase needs proper testing. Embracing automated testing can help identify and address potential issues, providing you with confidence in maintaining a robust and up-to-date Laravel application.
Keep packages up to date
Leveraging community packages simplifies our tasks, but it can expose us to potential vulnerabilities. For increased security, run composer updates regularly.
Eloquent ORM
In Laravel, Eloquent ORM (Object-Relational Model) offers a graceful Active Record implementation to manage your database efficiently. To ensure seamless functionality with the Eloquent ORM, adhering to the correct model naming convention is essential.
Every database table corresponds to a model within the Eloquent ORM, enabling you to interact with the database and execute diverse operations. Adhering to the convention of naming the model “User” for a table named “users” is crucial to prevent any potential Eloquent-related complications. By following this standard, you can make the most of Eloquent ORM’s capabilities and avoid any compatibility problems.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $table = 'users';
protected $fillable = ['name', 'email'];
}
=================================================================
// Creating a new user
User::create([
'name' => 'Tom Smith',
'email' => '[email protected]',
]);
// Finding a user by ID
$user = User::find(1);
// Updating a user's email
$user->update(['email' => '[email protected]']);
Use middleware instead of repeating code
In Laravel, leveraging middleware enables effective filtering or modification of the current request. They serve various purposes, including:
- Ensuring required permissions are met.
- Checking the user’s language and adjusting the locale accordingly.
As anticipated, Laravel provides a set of built-in middleware for essential tasks like authentication, rate limiting, and additional functionalities.
public function handle(Request $request, Closure $next) : Response
{
if (! $request->user()->hasEnoughTokens()) {
abort(403);
}
return $next($request);
}
get in touch to discuss your project
After your middleware performs its intended task, you have the flexibility to either block the request or allow it to proceed. This versatility empowers developers to manage incoming requests efficiently and tailor the application’s behavior based on specific conditions.
Use Eloquent naming conventions for table names
Laravel’s table naming conventions are not only user-friendly but also a crucial best practice that streamlines your team’s workflow.
When utilizing Artisan commands like php artisan make: model Post –migration –factory, Laravel takes care of the naming automatically, simplifying the process for you.
However, if you can’t utilize those commands, here’s a quick overview of the naming conventions:
For a model like “Post,” the recommended approach is to assign the table name as “posts,” employing the plural form (e.g., “remarks” for “Comment,” “responses” for “Reply,” etc.).
For a pivot table that links a “Post” to a “Comment” (e.g., “comment_post”):
Incorporate both table names.
Use the singular form.
Arrange them in alphabetical order.
By following these conventions, you ensure consistency and coherence within your Laravel project, promoting smoother collaboration among team members and simplifying database management tasks.
Read the documentation for more information.
Prevent N+1 issues with eager loading
Throughout our discussion of good practices, there’s still more to cover!
Have you ever come across the N+1 problem? If so, eager loading presents an excellent solution to mitigate its impact.
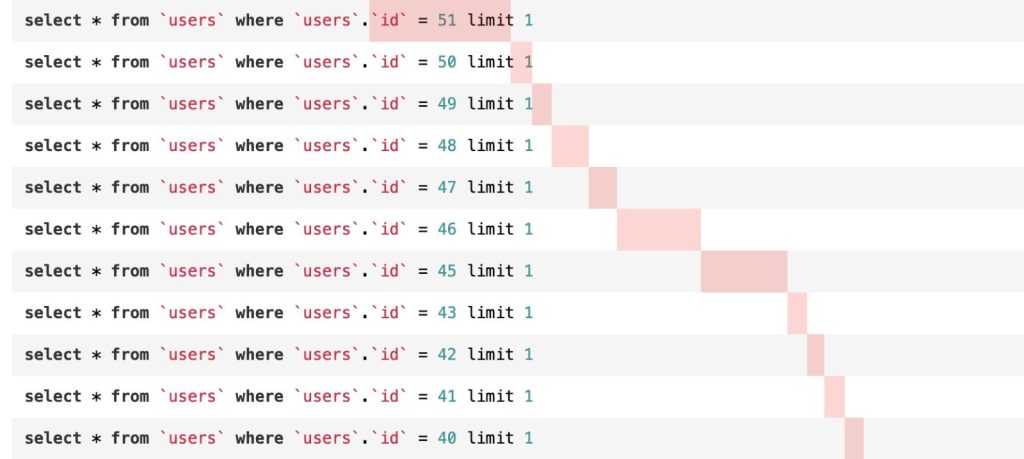
Imagine you’re showcasing a list of 30 posts along with their respective authors. By default, Eloquent would perform one query for the 30 posts and then an additional 30 queries, one for each author. This happens because the user relationship is lazily loaded, meaning it loads each time you call $post->user in your code.
To efficiently address this issue, you can easily resolve it by using the with() method. By doing so, you can significantly reduce the number of queries from 31 down to just 2, making your application much more optimized and responsive.
User::with('posts')->get();
To avoid N+1 problems, a practical approach is to raise exceptions whenever you attempt to lazy load any relationship. However, it’s essential to implement this restriction solely in your local environment, ensuring a smooth experience in production. By following this method, you can optimize your application’s performance and database queries, delivering a seamless user experience.
Model::preventLazyLoading(
! app()->isProduction()
);
Don’t track your .env file
Protecting sensitive information in your .env file is a crucial best practice that should never be overlooked, even if it may seem obvious to some. To ensure data security, refrain from tracking this file in version control (e.g., .gitignore).
If, by any chance, the .env file was mistakenly tracked in the past, take immediate action to safeguard your credentials. Update the sensitive information to new, secure credentials, preventing unauthorized access to your sensitive data from the Git history. By adhering to these measures, you can bolster your application’s security and protect your valuable information from potential threats.
Don’t track your compiled CSS and JavaScript
When working with Laravel, your CSS and JavaScript are typically generated from the original files located in the resources/CSS and resources/js directories. When you deploy your application to production, you have two options: either compile them on the server or create an artifact beforehand.
For those who are still using Laravel Mix, I strongly advise refraining from tracking these generated files in version control. It can become tedious to commit a new public/css/app.css or public/js/app.js every time a change is made.
To prevent this, you can easily add just two lines in your .gitignore file, effectively excluding these generated files from being tracked. This simple step ensures a cleaner version control history and streamlines your development process.
public/css
public/js
Add a Secure Layer of SSL and HTTPS
When developing an application, prioritizing security is paramount. One effective measure is the implementation of SSL certificates, which add encryption over the internet, safeguarding sensitive information. By employing SSL, data can be securely transmitted and decrypted by intended recipients, ensuring secure data exchanges. These best practices in Laravel enable businesses to execute queries in an unreadable format, enhancing data protection.
Another vital aspect is adopting HTTPS over HTTP for your website. By transitioning to HTTPS, you can secure crucial information, such as passwords and customer financial data, providing an added layer of protection. HTTPS also shields your website from potentially vulnerable attacks or data breaches, further bolstering the overall security posture of your application. Embracing these practices fosters a safe and trustworthy environment for users, instilling confidence in the security of your application.
Use of Autoload and Migrations
In Laravel, you have the flexibility to use service classes or plain PHP code as long as they can be autoloaded. This approach enhances script performance by preventing unnecessary checks for already loaded files. You no longer need to include the file each time it’s used in your code, as PHP handles it when required, resulting in an efficient and streamlined process.
Laravel Migrations offers a fantastic feature for version controlling your database. This allows your development team to seamlessly share and modify the application’s database schema. By frequently utilizing migrations in tandem with Laravel’s schema builder, you can swiftly create your web application’s database schema. It is advisable to avoid manual database creation and rely on Laravel Migrations, as it significantly eases the migration process, optimizing database management. Embracing these practices ensures a smooth and efficient development workflow for your Laravel projects.
Conclusion:
In conclusion, following these top 10 Laravel best practices is essential for developers aiming to create secure, efficient, and robust web applications. Keeping Laravel and packages up to date ensures enhanced security, improved performance, and access to the latest features. Adhering to Eloquent ORM naming conventions and using middleware effectively streamline database management and request handling. Preventing N+1 issues with eager loading optimizes application performance. Moreover, safeguarding sensitive information in the .env file and refraining from tracking it in version control bolsters data security. Avoiding the tracking of compiled CSS and JavaScript files in Git history simplifies the development process. Implementing SSL and HTTPS provides an added layer of protection for sensitive data. Finally, leveraging autoload and Laravel Migrations enhances script performance and enables seamless version control for database schema. Embracing these practices ensures a smooth and secure development process for Laravel projects, delivering exceptional user experiences.